No Extra Boxes, Please: When (and When Not) to Wrap Heap Data
If the compiler doesn’t force you to Box, you probably don’t need one!
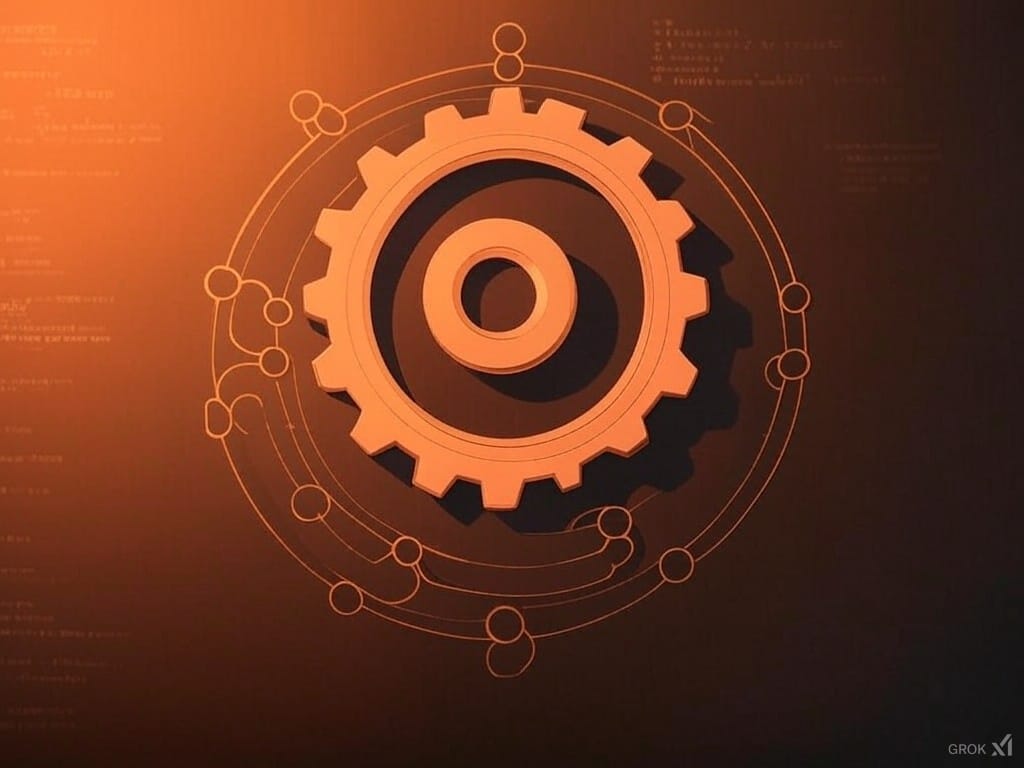
Rust often encourages us to think carefully about ownership, lifetimes, and memory allocation. Yet, it’s also very easy to throw a Box
around a heap-allocated value—even when you don’t need it.
I often come across the pattern of wrapping Vec<T>
, String
, and other heap-allocated types in a Box
. More often, wrapping these heap-allocated values inside a Box
is unnecessary unless you have a specific reason (e.g., trait objects, stable addresses in self‐referential structs, or an API that explicitly requires a Box
). In those redundant cases, you’d often be piling on an extra layer of indirection without any real performance benefit.
In this blog post, we’ll walk through many occasions using Box
is typically redundant, show some code examples, and highlight a few situations where you really would want a Box
.
Types like Vec<T>
and String
Are Already Heap-Allocated
When you do:
let v = vec![1, 2, 3];
let s = String::from("Hello!");
Under the hood, Rust stores three words on the stack for both Vec<T>
and String
:
- A pointer to the heap buffer
- The length
- The capacity
All the data—like the bytes of the string or the elements of the vector—lives on the heap. When you move (transfer ownership of) a Vec<T>
or String
to a function or another thread, Rust only copies that small metadata (pointer, length, capacity)—not the entire heap buffer. This is already an efficient way to pass large heap-allocated data around without incurring big copy overhead.
No Box
needed!
The Box Overhead
One might initially think:
“I’ll wrap my big vector in a Box
so it’s just a pointer on the stack!”
But consider what happens when you do:
let boxed_vec = Box::new(vec![1, 2, 3]);
Under the hood:
- You have one pointer on the stack (the
Box
itself). - That pointer leads to a heap-allocated
Vec<T>
, which contains:- Another pointer to the actual data elements
[1, 2, 3]
in yet another heap allocation (the vector’s buffer).
- Another pointer to the actual data elements
Effectively, you’ve introduced double indirection: each access to your final data now involves an extra pointer dereference. While this overhead is often small, it seldom improves performance. In fact, it may be slightly slower than just using a plain Vec<T>
, because you must chase an additional pointer to find the real data.
Unless you have a very specialized reason (for example, ensuring an absolutely stable Vec
pointer in certain self-referential or pinning scenarios), boxing an already-heap-allocated collection typically doesn’t speed up anything. Vec<T>
is already placing large data on the heap and moving it from function to function, which only copies a handful of metadata (pointer, length, capacity). There’s no big copy being avoided by adding Box
.
In short, if performance is your motivation, adding a box to a Vec
or String
generally isn’t helpful and can sometimes be counterproductive. For most use cases, a plain Vec
or String
is just fine.
When You Do Need Box<T>
(By Design)
1. Trait Objects: Box<dyn Trait>
If you have a function that expects a trait object (dynamic dispatch), you can’t just store it in a plain T
. You might need something like:
trait Processor {
fn process(&self);
}
struct PrintProcessor;
impl Processor for PrintProcessor {
fn process(&self) { println!("Processing..."); }
}
fn take_processor(p: Box<dyn Processor>) {
p.process();
}
fn main() {
let pr = PrintProcessor;
// Must create a box of a trait object
let p_boxed: Box<dyn Processor> = Box::new(pr);
take_processor(p_boxed);
}
Here, the size of dyn Processor
is unknown at compile time, so you need a pointer type that gives the compiler a notion of fixed information—Box<dyn Processor>
. If you tried Vec<dyn Processor>
or dyn Processor
by value, the compiler complains because it doesn’t know how much space to allocate. The box solves that by storing it on the heap with a fixed pointer size on the stack.
2. Recursive Types / Self-Referential Structures
A recursive type (e.g., a linked list) can’t be spelled out with a normal struct alone if it leads to an infinite size at compile time. For example:
enum Node {
Empty,
Cons(i32, Box<Node>), // <-- we need Box here
}
A singly linked list typically links to another node inside itself. Without a Box
, the compiler would try to compute the size of Node
as infinitely large. By boxing the nested Node
, you put that child node on the heap, bounding the size of Node
to something finite.
Concrete Example:
enum LinkedList {
Nil,
Cons(i32, Box<LinkedList>),
}
use LinkedList::*;
fn main() {
let list = Cons(1, Box::new(Cons(2, Box::new(Nil))));
// This compiles and works because the pointer in Box
// prevents an infinite size type.
}
Without that Box
, the compiler would complain about a recursive type having infinite size. That’s precisely the scenario where Rust forces you to use a pointer type (Box
, Rc
, or Arc
).
Code Examples
1. Passing a Vec<T>
by Value
fn consume_vec(v: Vec<u8>) {
println!("Got a vector of length: {}", v.len());
}
fn main() {
// A large vector (but stored as pointer+len+cap on the stack)
let big_vec = vec![42; 10_000_000];
// Passing by value copies only the pointer/length/capacity, not the entire 10 million elements
consume_vec(big_vec);
// `big_vec` is moved here, so we can't use it anymore in `main`
}
No Box
needed. Vec<T>
is already mostly on the heap. The function call is cheap in terms of what’s copied.
2.Using a Box<Vec<T>>
Instead (Likely Unnecessary)
fn consume_boxed_vec(bv: Box<Vec<u8>>) {
println!("Got a Boxed vec of length: {}", bv.len());
}
fn main() {
let big_vec = Box::new(vec![42; 10_000_000]);
consume_boxed_vec(big_vec);
// same behavior, but more pointer indirection
}
All we’ve really done is add an extra pointer hop. No real gain in performance or memory usage.
3.Valid Reasons: Trait Objects
trait Worker {
fn run(&self);
}
struct Logger;
impl Worker for Logger {
fn run(&self) {
println!("Logging something...");
}
}
fn execute_job(job: Box<dyn Worker>) {
job.run();
}
fn main() {
let my_job = Box::new(Logger);
execute_job(my_job);
}
Here, Box<dyn Worker>
is necessary for dynamic dispatch on Worker
. This is not an “unnecessary boxing” scenario.
Conclusion
Rust’s ownership and type system significantly optimize how data is stored and moved. Vec
, String
, and similar collections already place large data on the heap, so an extra Box
is usually not needed. However, certain language features—like trait objects or recursive types—require a pointer indirection to compile at all.
Key Takeaway:
- Don’t “box” your collection objects just to ensure they’re on the heap—they already do that internally.
- Reach for
Box<T>
only in the specialized cases where the compiler or design patterns demand it.
Keep your Rust code simpler, more direct, and free of unnecessary pointer nesting. More often, if the compiler doesn’t force you to use a Box
, you probably don’t need one!