Understanding map and filter_map in Rust: Handling Arrays with Option and Result Values
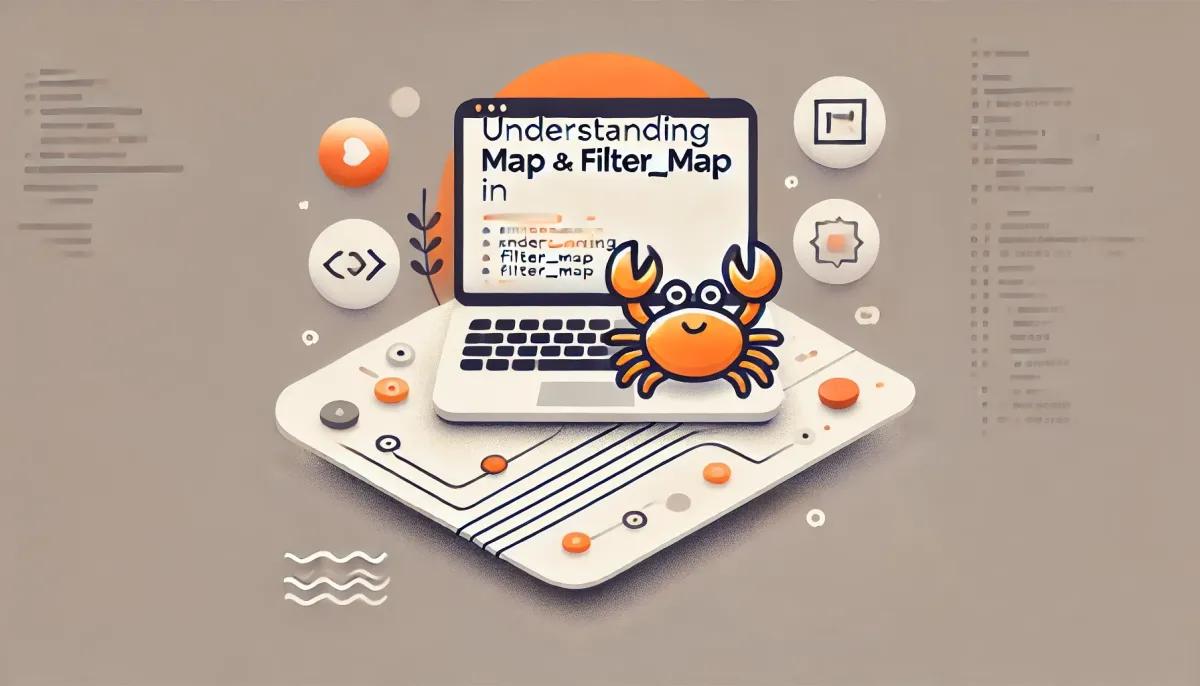
When working with collections in Rust programming language, especially arrays or vectors, it's common to encounter elements inside arrays wrapped in Option
or Result
types. Rust provides powerful iterator methods like map
and filter_map
to manipulate these arrays efficiently. In this blog post, we'll explore how map
and filter_map
behave when operating on arrays containing Option
and Result
values. We'll provide code examples and explanations to illustrate these concepts clearly.
Table of Contents
- Introduction to
map
andfilter_map
- Scenario 1: Arrays with Mixed
Option
Values - Scenario 2: Arrays with Mixed
Result
Values - When to Use
map
vs.filter_map
- Conclusion
Introduction to map
and filter_map
Before diving into specific scenarios, let's briefly understand what map
and filter_map
do in Rust.
map
: Applies a function to each element in an iterator, producing a new iterator with the results.filter_map
: Combines the functionality offilter
andmap
. It applies a function that returns anOption<T>
to each element. If the function returnsSome(value)
, that value is included in the new iterator. If it returnsNone
, the value is skipped.
Scenario 1: Arrays with Mixed Option
Values
Consider an array that contains a mix of Some
and None
values:
let numbers = [Some(1), None, Some(3), None, Some(5)];
Using map
with Option
Values
When we use map
on an iterator of Option
values, the function we provide is applied to each element, regardless of whether it's Some
or None
. However, since None
doesn't contain a value, we must handle it appropriately to avoid runtime errors.
Example:
let numbers = [Some(1), None, Some(3), None, Some(5)];
let incremented_numbers: Vec<Option<i32>> = numbers.iter().map(|num_option| {
match num_option {
Some(num) => Some(num + 1),
None => None,
}
}).collect();
println!("{:?}", incremented_numbers);
Output:
[Some(2), None, Some(4), None, Some(6)]
Explanation:
- We iterate over each
Option<i32>
innumbers
. - For
Some(num)
, we apply the functionnum + 1
and wrap the result back inSome
. - For
None
, we simply returnNone
.
Key Points:
- The output is a
Vec<Option<i32>>
, maintaining the same structure as the input. map
doesn't filter outNone
values; it processes each element individually.
Using filter_map
with Option
Values
filter_map
is useful when we want to transform only to Some
values and discard None
values.
Example:
let numbers = [Some(1), None, Some(3), None, Some(5)];
let incremented_numbers: Vec<i32> = numbers.iter().filter_map(|num_option| {
num_option.map(|num| num + 1)
}).collect();
println!("{:?}", incremented_numbers);
Output:
[2, 4, 6]
Explanation:
- We iterate over each
Option<i32>
innumbers
. num_option.map(|num| num + 1)
:- If
num_option
isSome(num)
, it returnsSome(num + 1)
. - If
num_option
isNone
, it returnsNone
.
- If
filter_map
only collects theSome
values, soNone
values are discarded.- The output is a
Vec<i32>
, containing only the incremented numbers.
Key Points:
filter_map
effectively filters outNone
values.- It allows us to work directly with the inner values of
Some
.
Scenario 2: Arrays with Mixed Result
Values
Now, let's consider an array containing Result
values, which may be Ok
or Err
.
let results = [Ok(1), Err("error"), Ok(3), Err("failed"), Ok(5)];
Using map
with Result
Values
Using map
on an iterator of Result
values applies the function to Ok
values and leaves Err
values unchanged.
Example:
let results = [Ok(1), Err("error"), Ok(3), Err("failed"), Ok(5)];
let incremented_results: Vec<Result<i32, &str>> = results.iter().map(|res| {
match res {
Ok(num) => Ok(num + 1),
Err(e) => Err(*e),
}
}).collect();
println!("{:?}", incremented_results);
Output:
[Ok(2), Err("error"), Ok(4), Err("failed"), Ok(6)]
Explanation:
- We iterate over each
Result<i32, &str>
inresults
. - For
Ok(num)
, we applynum + 1
and wrap the result back inOk
. - For
Err(e)
, we returnErr(e)
unchanged. - The output maintains the structure of
Result
, preservingErr
values.
Key Points:
map
does not filter outErr
values; they are passed through unchanged.- The function is only applied to
Ok
values.
Using filter_map
with Result
Values
We can use filter_map
along with the ok()
method to filter out' Err' values and only process' Ok' values.
Role of the ok()
Method
- The
ok()
method converts aResult<T, E>
into anOption<T>
.Ok(value).ok()
returnsSome(value)
.Err(_).ok()
returnsNone
.
Example:
let results = [Ok(1), Err("error"), Ok(3), Err("failed"), Ok(5)];
let incremented_values: Vec<i32> = results.iter().filter_map(|res| {
res.ok().map(|num| num + 1)
}).collect();
println!("{:?}", incremented_values);
Output:
[2, 4, 6]
Explanation:
- We iterate over each
Result<i32, &str>
inresults
. res.ok()
converts theResult
into anOption<i32>
:- For
Ok(num)
, it returnsSome(num)
. - For
Err(_)
, it returnsNone
.
- For
map(|num| num + 1)
is applied only toSome(num)
values.filter_map
collects the resultingSome
values, discardingNone
.- The output is a
Vec<i32>
containing only the incremented numbers fromOk
values.
Key Points:
filter_map
withok()
filters outErr
values.- Only
Ok
values are transformed and collected. - The
ok()
method is essential in convertingResult
toOption
for this use case.
When to Use map
vs. filter_map
Understanding the behavior of map
and filter_map
is crucial for writing concise and safe Rust code.
- Use
map
when:- You want to apply a function to every element in an iterator.
- You need to preserve the structure of the original collection, including
None
orErr
values. - Example: Transforming values but keeping track of missing data or errors.
- Use
filter_map
when:- You want to both filter and transform elements in a single operation.
- You're only interested in the
Some
orOk
values and wish to discardNone
orErr
values. - Example: Extracting valid results and ignoring failures.
Conclusion
Rust's iterator methods map
and filter_map
provide powerful ways to process collections containing Option
and Result
types. You can write more efficient and expressive code by understanding how these methods interact with Some
, None
, Ok
, and Err
values.
map
: Transforms each element individually, preserving the collection's structure.filter_map
: Filters out unwanted elements (None
orErr
) and applies transformations to the rest.
Using these methods appropriately allows you to handle complex data transformations easily, leading to cleaner and more maintainable code.
Happy coding in Rust!