Simplifying Rust Lifetimes: When Owning Data Reduces Complexity
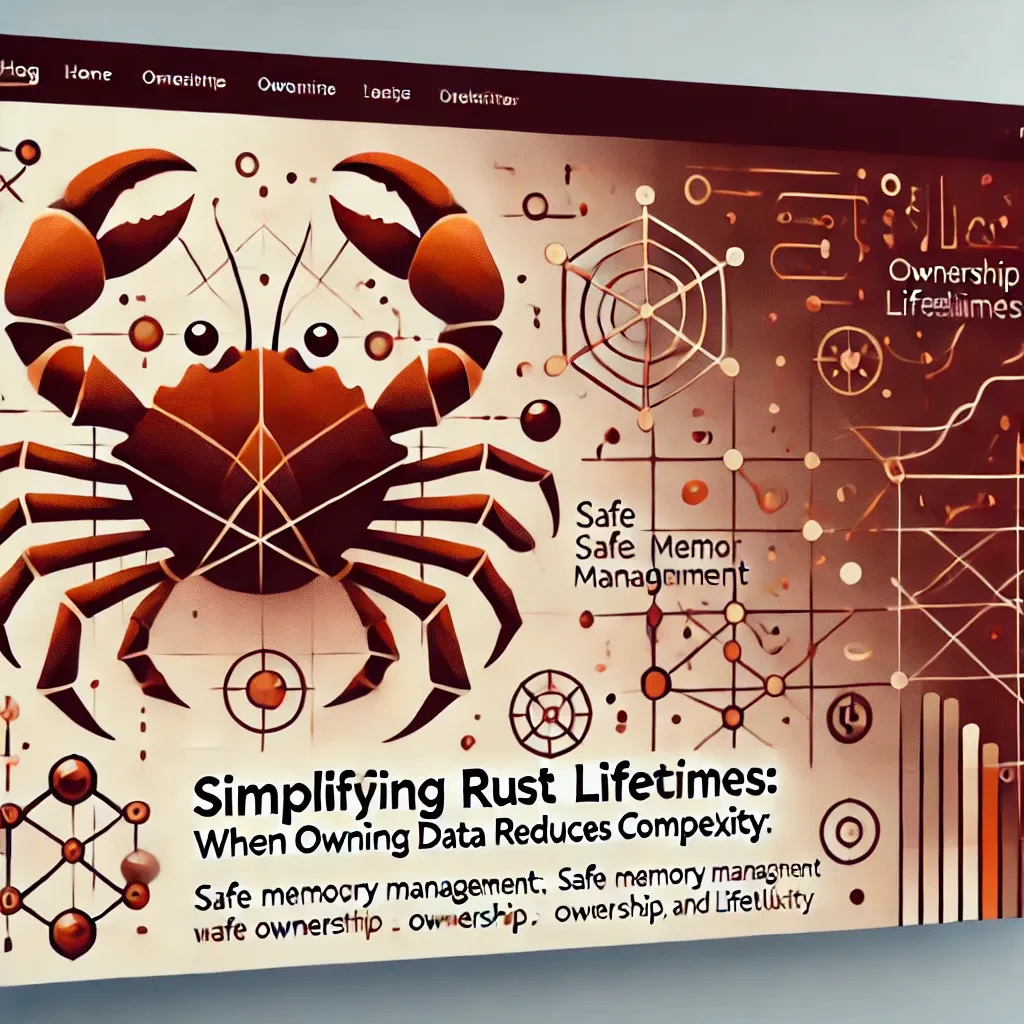
In Rust programming language, lifetimes are a powerful feature that ensures memory safety without needing a garbage collector. However, they can sometimes introduce complexity, mainly when dealing with borrowed references in structs and functions. This complexity can spread throughout your codebase, making it harder to read and maintain.
This post explores how owning data instead of borrowing references can simplify code and reduce the complexity caused by lifetimes in certain circumstances. We'll walk through an example demonstrating how borrowing can complicate things and how changing to owning data can make the code easier to manage.
The Problem with Borrowed References and Lifetimes
Consider a simple scenario where we have a struct that holds a reference to a string slice:
struct Message<'a> {
content: &'a str,
}
impl<'a> Message<'a> {
fn print(&self) {
println!("{}", self.content);
}
}
Here, the Message
struct holds a borrowed reference to a string slice &'a str
. The lifetime 'a
ensures that the reference content
is valid as long as the Message
instance exists.
Propagation of Lifetimes
Now, suppose we have a function that creates a Message
and returns it:
fn create_message<'a>(text: &'a str) -> Message<'a> {
Message { content: text }
}
Any function that calls create_message
and uses the returned Message
must also manage the lifetime 'a
. This lifetime parameter can start to propagate through your codebase.
For example:
fn main() {
let text = String::from("Hello, Rust!");
let msg = create_message(&text);
msg.print();
}
In this simple case, the lifetime is managed implicitly, and the compiler can infer it. However, as your code grows and you introduce more structs and functions that depend on Message<'a>,
you might find yourself adding lifetime annotations throughout your code, increasing complexity.
Adding More Complexity
Now imagine introducing another struct that holds a Message
:
struct Envelope<'a> {
message: Message<'a>,
}
impl<'a> Envelope<'a> {
fn deliver(&self) {
self.message.print();
}
}
With this addition, Envelope
also requires the lifetime 'a
, and any function that uses Envelope
must also handle the lifetime.
For example:
fn send_envelope<'a>(env: Envelope<'a>) {
env.deliver();
}
As you can see, lifetimes spread across your codebase, adding more complexity as structs and functions depend on each other.
Simplifying by Owning Data
One way to reduce this complexity is to have Message
own the data instead of borrowing a reference. Here's how we can modify the Message
struct:
struct Message {
content: String,
}
impl Message {
fn print(&self) {
println!("{}", self.content);
}
}
Now, Message
owns a String
instead of borrowing a &str
. This change eliminates the need for lifetime annotations in Message
.
Our create_message
function becomes:
fn create_message(text: &str) -> Message {
Message {
content: text.to_string(),
}
}
This version of the function no longer has lifetime parameters. We can now use create_message
in main
without worrying about lifetimes:
fn main() {
let text = String::from("Hello, Rust!");
let msg = create_message(&text);
msg.print();
}
Updating Envelope
We can update Envelope
similarly to owning its Message
:
struct Envelope {
message: Message,
}
impl Envelope {
fn deliver(&self) {
self.message.print();
}
}
fn send_envelope(env: Envelope) {
env.deliver();
}
Again, no lifetimes are needed here, making the code easier to read and maintain.
Trade-offs of Owning Data vs. Borrowing
When to Borrow References
- Large Data: Cloning large data structures can be expensive regarding performance and memory usage.
- Shared Access: Borrowing is appropriate if multiple parts of your code need to access the same data without transferring ownership.
When to Own Data
- Small Data: Cloning small types like strings or numbers has minimal overhead.
- Simplify Lifetimes: Owning data removes the need for lifetime annotations, making your code easier to read and maintain.
Conclusion
Lifetimes are an essential feature in Rust that ensures memory safety. However, they can add complexity when borrowed references propagate through your structs and functions. In many cases, especially when working with small types like strings, owning the data can simplify the code by eliminating lifetime annotations. This approach can reduce cognitive load and make the code easier to maintain while benefiting from Rust's memory safety guarantees.
Key Takeaways
- Assess Data Size: Consider owning data to simplify lifetimes for small data types.
- Balance Trade-offs: While owning data can increase memory usage, the trade-off may be worth it for cleaner code, especially when dealing with primary types.
- Simplify APIs: Owning data can simplify your APIs, as consumers don't need to worry about lifetimes.
By carefully choosing when to borrow and when to own data, you can write robust, safe, and maintainable code without unnecessary complexity from lifetimes.